So I set out to make the LED blink a merry blink tonight, but was finally thwarted when I came to the realisation I’d need UV light to erase the EPROMs to load my code in. Suffice to say this was mighty frustrating.
I’m getting ahead of the story, so let’s start from the beginning
The beginning
Starting tonight with a goal to have “something” running on this board before I went to bed I started sorting out my development environment. Setting up the required software and tools to get the job done so to speak.
I installed all the GNU m68k tools. I made a nice little Makefile to assemble my assembly…
M68K=m68k-linux-gnu
AS=$(M68K)-as
LD=$(M68K)-ld
DUMP=$(M68K)-objdump
START=0x00
FMT=binary
#FMT=ihex
CPU=-m68000
SRCS=blink.s
OBJS=$(SRCS:.s=.o)
MAIN=blink.bin
.PHONY: dump clean
all: $(MAIN)
@echo Builds blink.bin
$(MAIN): $(OBJS)
$(LD) -e $(START) --oformat $(FMT) -o $(MAIN) $(OBJS)
.s.o:
$(AS) $(CPU) -o $@ $<
clean:
rm *.o $(MAIN)
dump: $(MAIN)
$(DUMP) $(CPU) --disassemble-all --target=$(FMT) --start-address=$(START) $(MAIN)
I even bashed together a little test program to twiddle the LED bit of the 74LS259…
/*
* Blink LED connected to 74LS259 output.
*
* ROM is at 0x00000-0x0FA00.
* RAM is at 0x20000-0x2FA00
* 74LS259 is at 0x60000.
*/
.equ PORT, 0x60000
/* Iterations of delay loop before updating output value */
.equ DELAY, 50000
.org 0
/* set initial SSP (supervisor stack pointer) */
.byte 0, 0, 0, 0
/* set the program counter */
.byte 0, 0, 4, 0
.org 0x400
move.l #PORT, %a0
.top:
move.b #0x0E, %d0 /* set Q7 to low in the addressable latch mode */
move.b %d0, (%a0) /* write to 'LS259 */
move.l #DELAY, %d1
.delay1:
tst.l %d1
beq .delay1end
subq.l #1, %d1
jmp .delay1
.delay1end:
move.b #0x0F, %d0 /* set Q7 to high in the addressable latch mode */
move.b %d0, (%a0) /* write to 'LS259 */
move.l #DELAY, %d1
.delay2:
tst.l %d1
beq .delay2end
subq.l #1, %d1
jmp .delay2
.delay2end:
jmp .top
.org 0xFFFA
jmp .top
And like a late night TV shopping channel ad I’ll even throw in a free set of steak knives tool to split the resulting binary into 32KB interleaved chunks so they’d work on the board.
#!/usr/bin/python3
import os
import argparse
parser = argparse.ArgumentParser()
parser.add_argument("src")
args = parser.parse_args()
even_fn = "even-{}".format(args.src)
odd_fn = "odd-{}".format(args.src)
with open(args.src, 'rb') as src:
with open(even_fn, 'wb') as even:
with open(odd_fn, 'wb') as odd:
src.seek(0, os.SEEK_END)
src_size = src.tell()
src.seek(0)
for c in range(0, src_size):
src.seek(c)
if c % 2:
odd.write(src.read(1))
else:
even.write(src.read(1))
Also buy one get one free; I upgraded the software for the Minipro TL866 on OSX. I even figured out the magic device type for my EPROMs.
Then it happened…
I went and tried to write the first “even” binary to the EPROM…
$ minipro -p "27C256 @DIP28 #2" -w even-blink.bin
Found Minipro TL866A v03.2.72
Chip ID OK: 0x298c
Writing Code... OK
Reading Code... OK
Verification failed at 0x200: 0x20 != 0x00
COMPUTER SAYS NO...
Beware, losing all hope…
Damn it! I forgot I need to erase these things first.
Hunting around the garage for any source of UV I knew my search was futile. I even resorted to madness when I found a big bag of “UV” LEDs.
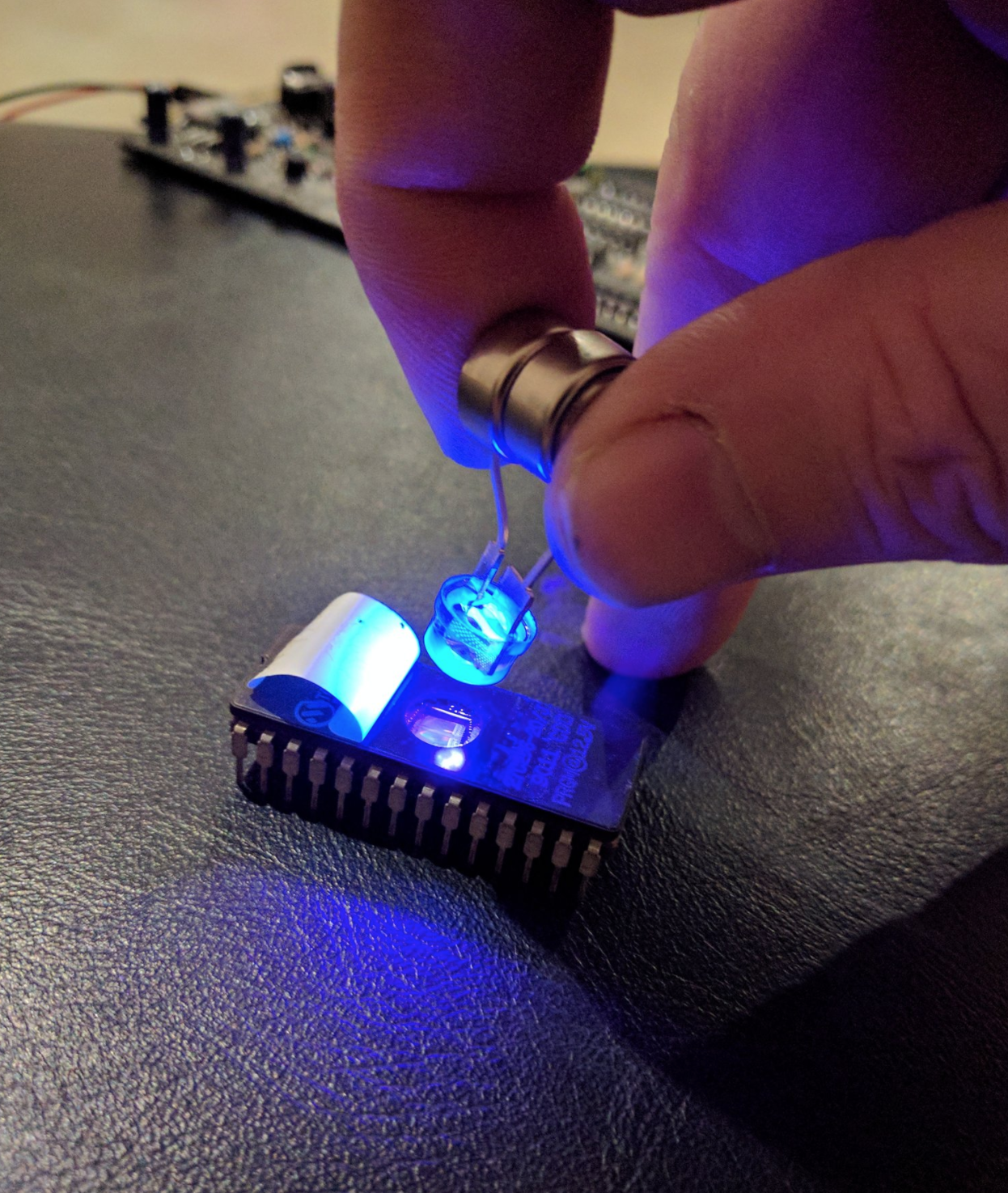
So, now what…
Well I’ll need to figure out a source of UV. However I also figured I’d just buy some newer flash based EEPROMs in the mean time. Element14 have them in stock locally and I should see them before the weekend hopefully.
I ordered two AT28C256s, which are 98% pin compatible and fast enough for this board. I need to hack a pin swap between pin 1 and 27, but that’s a small price to pay I guess.
Still very disappointed, and my wife is likely to kill me when I sneak into bed at 1:00am. Wouldn’t be so bad if I had the taste of victory instead of the bitter pill of defeat.
I put up a good fight.
But UV got the better of me.
Here’s to tomorrow!
Comments